Map of Trieste
Category: geographical
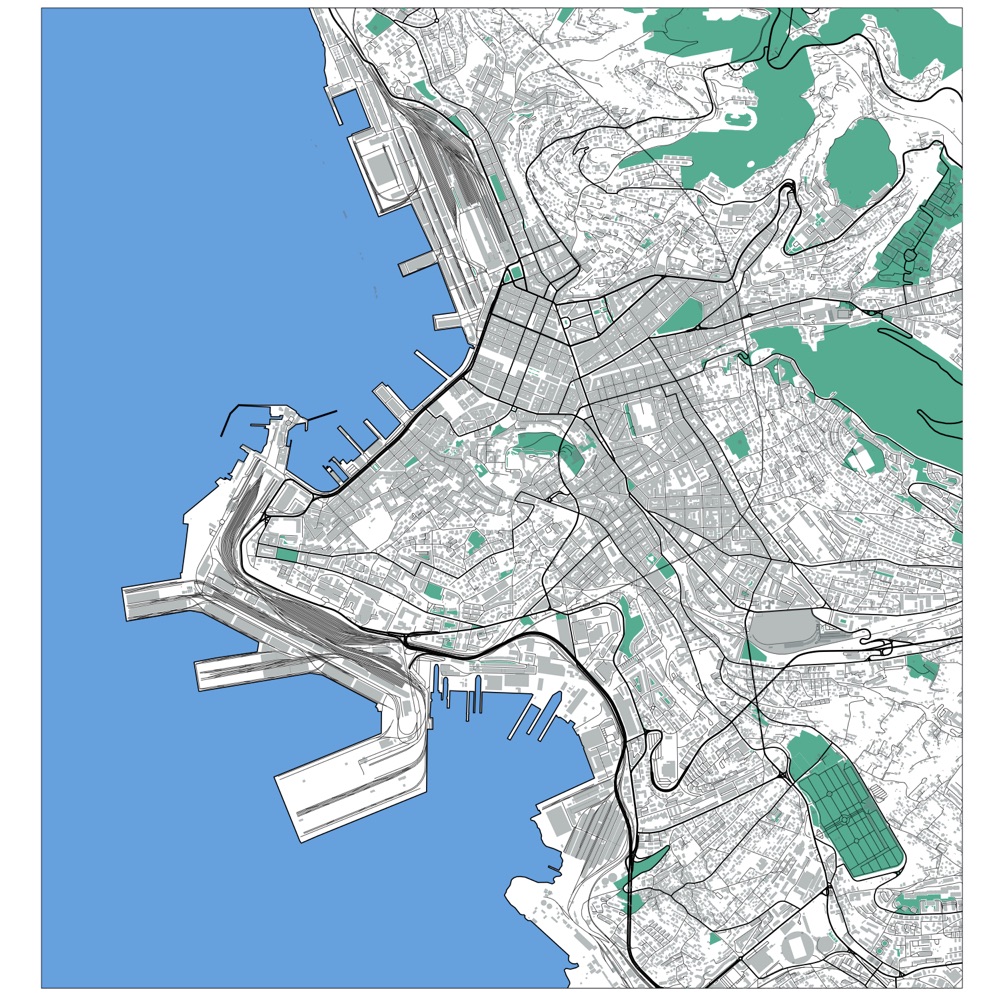
Light version
library(sf)
library(foreign)
library(tidyverse)
library(lwgeom)
library(stringi)
options(stringsAsFactors = FALSE)
# download SHP files for region, e.g. from Geofabrik
# http://download.geofabrik.de/europe/italy/nord-est.html
buildings_a <- read_sf('gis_osm_buildings_a_free_1.shp')
landuse_a <- read_sf('gis_osm_landuse_a_free_1.shp')
natural <- read_sf('gis_osm_natural_free_1.shp')
natural_a <- read_sf('gis_osm_natural_a_free_1.shp')
railways <- read_sf('gis_osm_railways_free_1.shp')
roads <- read_sf('gis_osm_roads_free_1.shp')
traffic <- read_sf('gis_osm_traffic_free_1.shp')
traffic_a <- read_sf('gis_osm_traffic_a_free_1.shp')
transport <- read_sf('gis_osm_transport_free_1.shp')
transport_a <- read_sf('gis_osm_transport_a_free_1.shp')
water_a <- read_sf('gis_osm_water_a_free_1.shp')
waterways <- read_sf('gis_osm_waterways_free_1.shp')
# download the country boundaries as SHP file
# https://wambachers-osm.website/boundaries/
# we need it once with the border along the land area and once inside the water
# because the sea is not annotated in any of the other SHP files and if we draw
# the background blue, there will also be a blue border around the whole map.
# this trick is only possible because we have zoomed into the map far enough
# that all the parts of the sea seen in it still belong to Italy and therefore,
# when downloading the border of Italy including the water, we can use that to
# draw the sea and then the land on top
country_boundaries <- read_sf('country_boundaries/Italy_AL2.shp')
country_boundaries_with_water <- read_sf('country_boundaries_with_water/Italy_AL2.shp')
coordinates_box <- c(
xmin = 13.738060,
ymin = 45.620521,
xmax = 13.804836,
ymax = 45.670204
)
box <- list(
coordinates = coordinates_box,
buildings_a = st_crop(buildings_a, coordinates_box),
landuse_a = st_crop(landuse_a, coordinates_box),
natural = st_crop(natural, coordinates_box),
natural_a = st_crop(natural_a, coordinates_box),
railways = st_crop(railways, coordinates_box),
roads = st_crop(roads, coordinates_box),
traffic = st_crop(traffic, coordinates_box),
traffic_a = st_crop(traffic_a, coordinates_box),
transport = st_crop(transport, coordinates_box),
transport_a = st_crop(transport_a, coordinates_box),
water_a = st_crop(water_a, coordinates_box),
waterways = st_crop(waterways, coordinates_box)
country_boundaries = st_crop(country_boundaries, coordinates_box),
country_boundaries_with_water = st_crop(country_boundaries_with_water, coordinates_box)
)
# makes it easier to come back to it later
saveRDS(box, 'box.rds')
blankbg <- theme(
axis.line = element_blank(),
axis.text.x = element_blank(),
axis.text.y = element_blank(),
axis.ticks = element_blank(),
axis.title.x = element_blank(),
axis.title.y = element_blank(),
panel.background = element_blank(),
panel.border = element_blank(),
panel.grid.major = element_blank(),
panel.grid.minor = element_blank(),
plot.background = element_blank(),
)
p <-
ggplot() +
geom_sf(data = box$country_boundaries_with_water, color = 'black', size = 0.35, fill = '#78b1e4') +
geom_sf(data = box$country_boundaries, color = 'black', size = 0.35, fill = 'white') +
geom_sf(data = box$water_a %>% dplyr::filter(fclass %in% c('water')), size = 0, fill = '#78b1e4') +
geom_sf(data = box$landuse_a %>% filter(fclass %in% c('grass','cemetery','forest','park')), size = 0, fill = '#65b8a2') +
geom_sf(data = box$roads %>% dplyr::filter(fclass %in% c('unclassified','service','residential')), color = 'black', size = 0.15) +
geom_sf(data = box$railways, size = 0.15, color = 'black') +
geom_sf(data = box$roads %>% dplyr::filter(fclass %in% c('primary','primary_link','motorway','motorway_link','trunk','trunk_link')), color = 'black', size = 0.6) +
geom_sf(data = box$roads %>% dplyr::filter(fclass %in% c('secondary','secondary_link')), color = 'black', size = 0.5) +
geom_sf(data = box$roads %>% dplyr::filter(fclass %in% c('tertiary','tertiary_link')), color = 'black', size = 0.4) +
geom_sf(data = box$buildings_a, color = '#7f8c8d', alpha = 0.5, size = 0.05, fill = '#7f8c8d') +
blankbg +
theme(plot.background = element_rect(fill = 'white', color = NA)) +
coord_sf(xlim = box$coordinates[c(1,3)], ylim = box$coordinates[c(2,4)], expand = FALSE)
ggsave('trieste_light.png', p, scale = 1, width = 10, height = 10, units = 'in', dpi = 150)
Dark version
library(sf)
library(foreign)
library(tidyverse)
library(lwgeom)
library(stringi)
options(stringsAsFactors = FALSE)
# load from previous code block
box <- readRDS('box.rds')
blankbg <- theme(
axis.line = element_blank(),
axis.text.x = element_blank(),
axis.text.y = element_blank(),
axis.ticks = element_blank(),
axis.title.x = element_blank(),
axis.title.y = element_blank(),
panel.background = element_blank(),
panel.border = element_blank(),
panel.grid.major = element_blank(),
panel.grid.minor = element_blank(),
plot.background = element_blank(),
)
p <-
ggplot() +
geom_sf(data = box$country_boundaries_with_water, color = 'black', size = 0.35, fill = '#2d5272') +
geom_sf(data = box$country_boundaries, color = 'black', size = 0.35, fill = '#2c3e50') +
geom_sf(data = box$water_a %>% dplyr::filter(fclass %in% c('water')), size = 0, fill = '#2d5272') +
geom_sf(data = box$landuse_a %>% filter(fclass %in% c('grass','cemetery','forest','park')), size = 0, fill = '#183b32') +
geom_sf(data = box$roads %>% dplyr::filter(fclass %in% c('unclassified','service','residential')), color = '#6d5f43', size = 0.15) +
geom_sf(data = box$railways, size = 0.15, color = '#6d5f43') +
geom_sf(data = box$roads %>% dplyr::filter(fclass %in% c('tertiary','tertiary_link')), color = '#c39c30', size = 0.4) +
geom_sf(data = box$roads %>% dplyr::filter(fclass %in% c('secondary','secondary_link')), color = '#d9ac25', size = 0.5) +
geom_sf(data = box$roads %>% dplyr::filter(fclass %in% c('primary','primary_link','motorway','motorway_link','trunk','trunk_link')), color = '#d9ac25', size = 0.6) +
geom_sf(data = box$buildings_a, color = '#cb862b', alpha = 0.25, size = 0.05, fill = '#7c5c3f') +
blankbg +
theme(plot.background = element_rect(fill = '#2c3e50', color = NA)) +
coord_sf(xlim = box$coordinates[c(1,3)], ylim = box$coordinates[c(2,4)], expand = FALSE)
ggsave('trieste_dark.png', p, scale = 1, width = 10, height = 10, units = 'in', dpi = 150)
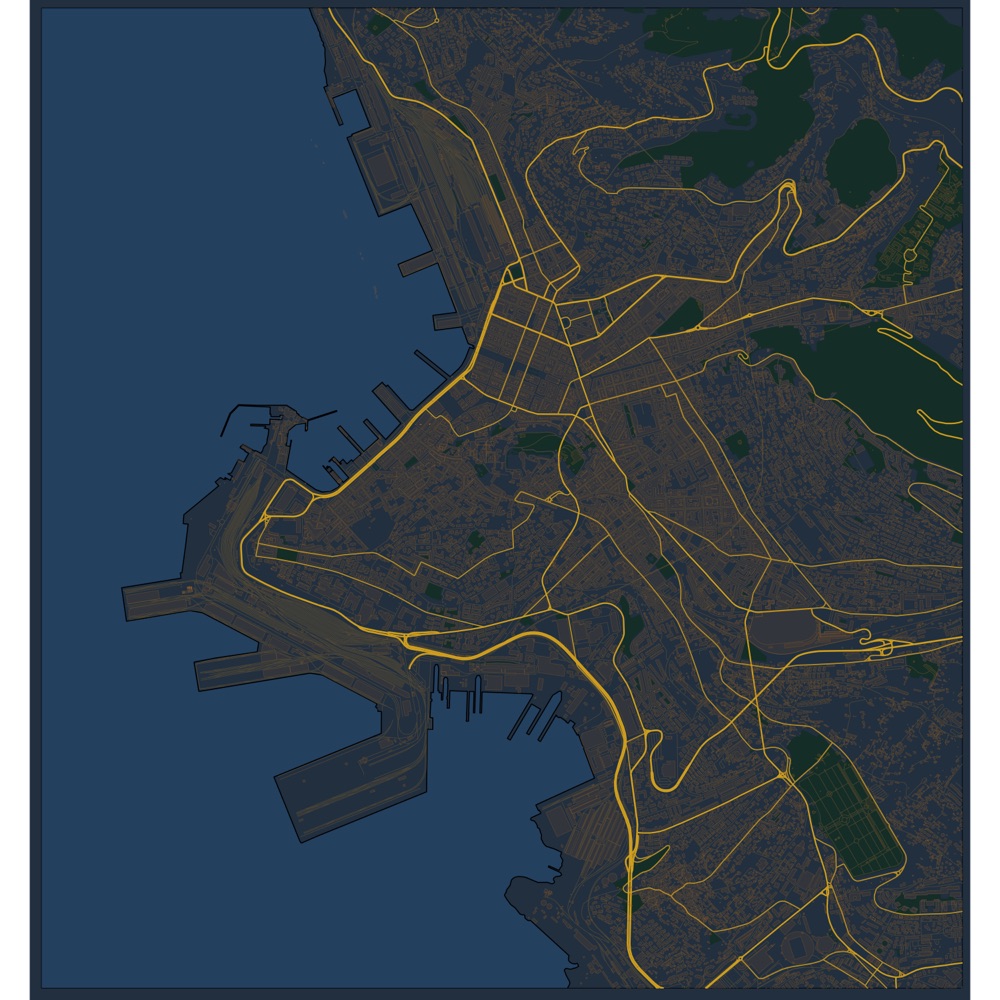